Secure passwords are absolutely essential in today’s age. Although it may seem obvious to those who are thoroughly versed in cybersecurity, millions of people use the same, insecure password for many profiles and accounts.
A random password generator is a great way to make sure that secure, random, and sophisticated passwords are being generated.
In order to do this, let’s start by importing the characters that we will be using for the password. This includes numbers, letters, and punctuation.
We will also be importing the module “secrets”. While the module “random” could be used to create the same result that we are looking for, it’s important to remember that we need to be generating cryptographically strong passwords. That being said, the “secrets” module is superior for generating truly random values.
import string
import secrets
Next, we will welcome the user and define the range of characters that we will be using for our program.
print("Hello! Welcome to the Password Generator. ")
lowercaseLetters = string.ascii_lowercase
uppercaseLetters = string.ascii_uppercase
numbers = string.digits
specialCharacters = string.punctuation
alphabet = lowercaseLetters + uppercaseLetters + numbers + specialCharacters
Our alphabet is now defined by upper and lower case letters, numbers, and punctuation marks.
Next, we will allow the user to input a number that will be the total length of the password:
length = int(input("Desired password character length: "))
Finally, we need to create a loop function that joins together a random sequence of letters, numbers, and punctuation at the length given by the user. We do this by creating a loop using the length given and combining the resulting characters:
while True:
result = ''
for i in range(length):
result += ''.join(secrets.choice(alphabet))
It’s important to remember that the password we are generating is going to be used by humans. Humans prefer certain aspects when it comes to passwords, typically that there is not a large amount of punctuation.
Also, certain parameters are required when creating a password for certain services. These include a mandatory minimum of punctuation, numbers, and uppercase letters.
With all of this being considered, we need to add parameters to our generator to make sure that the passwords we are generating meet these parameters. We do this by limiting the number of punctuation and number characters to 2 each, and by making sure that there is at least 1 uppercase letter.
if (sum(char in specialCharacters for char in result) == 2 and
sum(char in numbers for char in result) == 2 and sum(char in uppercaseLetters for char in result) == 1):
break
By adding these parameters, we ensure that the password allows for easy input by humans, while also meeting the complexity requirements mandated by password creation services.
Finally, we need to print our password:
print("Here's your secure password: ", result)
For a desired password length of 12 characters, the generator created the following password:
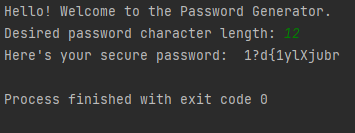
How do we know that this password is secure? To make sure that the password is secure, I input the password into a password strength detector.

Using python, we were able to generate a secure password that would allow for relatively easy input by the user.
This method can be used by all users who are concerned about their password security. To allow for easy recollection, it’s recommended that a user utilizes a password manager, such as Bitwarden, Norton Password Manager, or Keeper in conjunction with the secure password generator.